In this post, let's see how we can create/update Azure API Management APIs
from both classic release pipelines and YAML based pipelines.
Here I am assuming, We have already created Azure API Management Service in
Azure and we have set up Products.
First, let's have a look at how we can use this in classic release pipelines.
Once this extension is installed, you should be able to see a bunch of tasks
starting with API Management - *. From there what we need is
API Management - Create or Update API task. We just need to add this
task after the deployment of the API.
|
API Management - Create or Update API
|
Then it's just a matter of configuring the task.
|
API Management - Create or Update API Settings
|
Most of the things here are pretty obvious, so I am not going to explain
each one of them. The important ones are,
- Products
-
Since I have already created a product in the APIM, I can just enter
the name of the Product.
-
If you are creating the Product as part of the pipeline (using
API Management - Create or update product task), then you need
to select Product created by previous task.
- OpenAPI Specification
-
The APIM needs to have access to OpenAPI specification in order to
create the API. You have several options here. You need to select the
version, it's format (json or yaml), any authorization needed to
access the specification and the location (URL, Code or Build
Artifact)
- Configuration of the API
-
Here, the nice thing is you can specify the Policy. I prefer
maintaining the policy in the code, so here I have just selected my
policy by providing it's path.
So that's basically it. Once the API is deployed, this task will run, it
will pull down the OpenAPI specification, create/update APIM API, apply the
policy and it's all good to go.
If you prefer having deployment steps as part of YAML pipeline, we can
easily set up this task there as well. Basically something like this.
- task: stephane-eyskens.apim.apim.apim@5
displayName: 'API Management - Create/Update API '
inputs:
ConnectedServiceNameARM: ''
ResourceGroupName: ''
ApiPortalName: ''
UseProductCreatedByPreviousTask: false
product1: ''
OpenAPISpec: 'v3'
swaggerlocation: ''
targetapi: ''
DisplayName: ''
pathapi: ''
subscriptionRequired: false
TemplateSelector: Artifact
policyArtifact: '$(Pipeline.Workspace)\scripts\apim\apim-policy.xml'
MicrosoftApiManagementAPIVersion: '2018-01-01'
Don't worry, you have the UI to select the options just like classic releases.
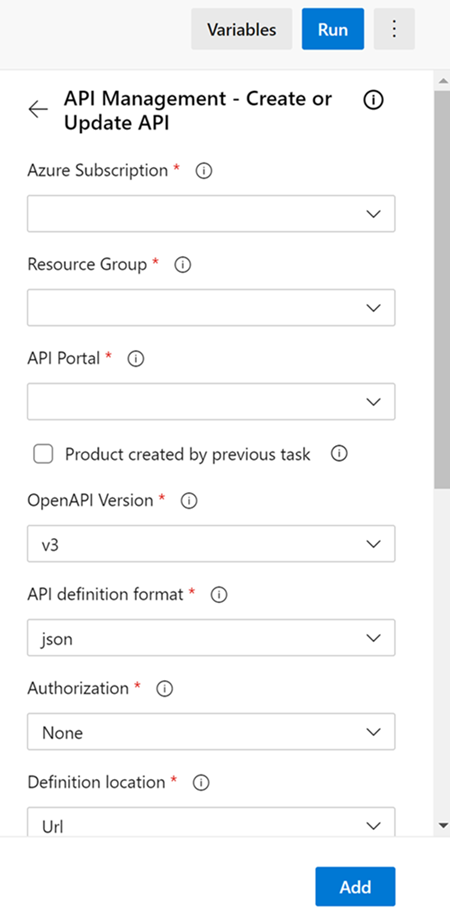 |
API Management - Create or Update API Settings |
So hope this helps.
Happy Coding.
Regards,
Jaliya