In this post let's see how we can setup configuration settings for ASP.NET Core Application to read from
Azure App Configuration.
Azure App Configuration is a managed service by Azure that provides a way to centrally manage application settings and feature flags. Nowadays a single application consists of multiple components running in different places, so the idea behind
Azure App Configuration is having a single place to manage all your configuration settings.
It's always better to go by demonstration. Let's start by creating an Azure App Configuration service in Azure.
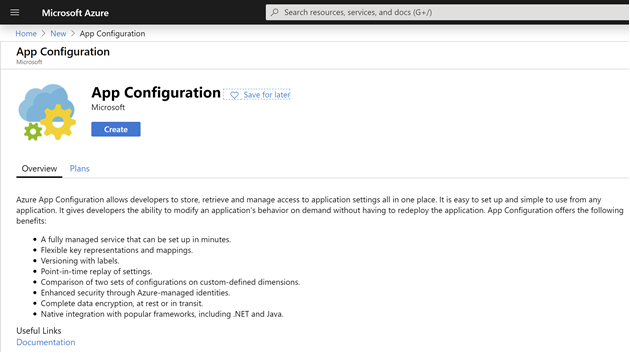 |
App Configuration |
I am clicking on create.
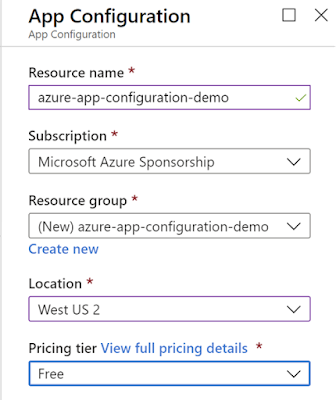 |
App Configuration Setup |
We just need to enter the basic information and I am completing the creation with the above settings.
Once that is created, we can create the settings by navigating into
Settings ->
Configuration Explorer.
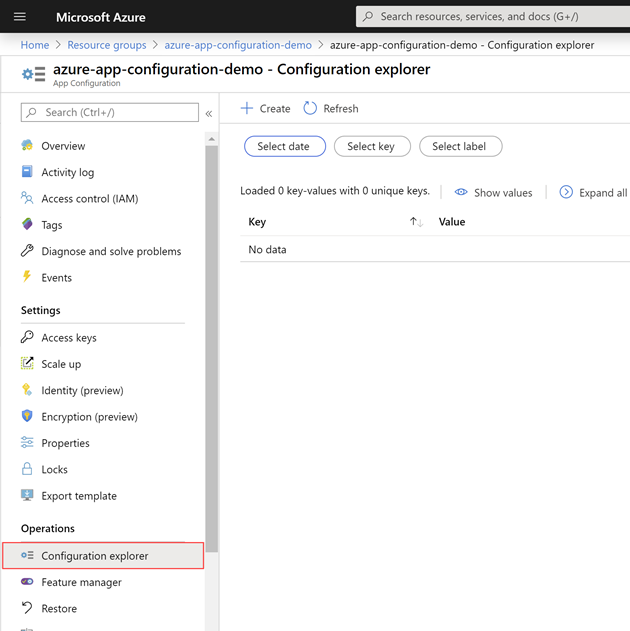 |
Configuration explorer |
Configuration settings here are basically a Key-Value pair. Before adding any values, let's create an ASP.NET Core Application and configure it to read settings from here. For the demo purpose, I will just create an API application named
AzureAppConfigurationDemo and I am adding the NuGet package
Microsoft.Azure.AppConfiguration.AspNetCore which will enable adding Microsoft Azure App Configuration as a configuration source in our application.
Now I am updating the Program.cs to configure the Azure App Configuration we created above to be used in our application.
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder
.ConfigureAppConfiguration((hostingContext, config) =>
{
var settings = config.Build();
config.AddAzureAppConfiguration(settings["ConnectionStrings:AppConfig"]);
})
.UseStartup<Startup>();
});
}
Here, under the
ConfigureAppConfiguration, we can add the AzureApp Configuration with a target connection string. You can find the connection string under Settings -> Access Keys inside the Azure App Configuration.
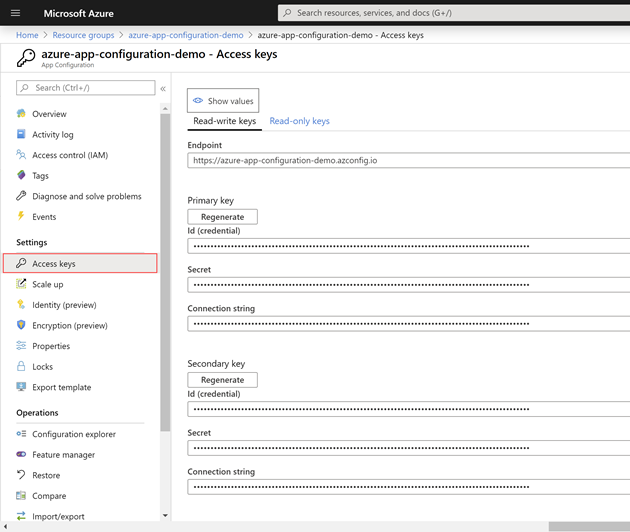 |
Access Keys |
Now I am adding the secret named
ConnectionStrings:AppConfig to Secret Manager. We can do this by either running
dotnet user-secrets set command or if you are using Visual Studio by right-clicking on the project and
Manage User Secrets.
dotnet user-secrets set ConnectionStrings:AppConfig "<ConnectionString>"
The secret Manager is used only to test things locally. When the app is deployed to Azure App Service, for example, we can use the Connection Strings application setting in App Service.
In my application, I have below dummy controller, which will return the value of a key named "
AzureAppConfigurationDemo:TestKey" inside the configuration settings
[ApiController]
[Route("[controller]")]
public class SettingsController : ControllerBase
{
private IConfiguration Configuration { get; }
public SettingsController(IConfiguration configuration)
{
Configuration = configuration;
}
public IActionResult GetValue()
{
string value = Configuration["AzureAppConfigurationDemo:TestKey"];
return Ok(value);
}
}
Now let's create the key: "
AzureAppConfigurationDemo:TestKey" by going back to
Settings ->
Configuration Explorer in our Azure App Configuration.
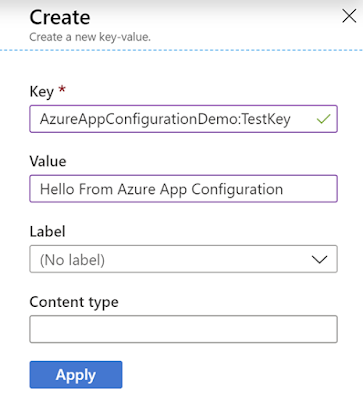 |
Add Key |
I am leaving Label and Content Type empty for now and I am clicking on Apply.
Now we are all set. Let's run the application.
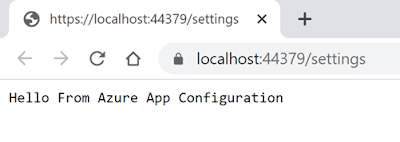 |
Result |
And here it is. Hope this helps.
Happy Coding.
Regards,
Jaliya