And with these, we get the opportunity to try out some of the features which will be coming in C# 8.0. In this post, let’s go through Nullable Reference Types which is one of them.
Without explaining the feature, let’s consider the following code.
class Program
{
static void Main(string[] args)
{
Employee employee = null;
Console.WriteLine(employee.Name);
}
}
class Employee
{
public string Name { get; set; }
}
The code is pretty obvious, you can see that when the employee is null, trying to access its property Name will throw a NullReferenceException.
Now closely look at the following two pictures. First one is when we are on
C# 7.3 (Visual Studio 15.9.3) and the second one is when we are on
C# 8.0 (Visual Studio 16.0.0 Preview 1.0). If you are wondering how to select language version in Visual Studio, check out this
post.
 |
C# 7.3 (Visual Studio 15.9.3) |
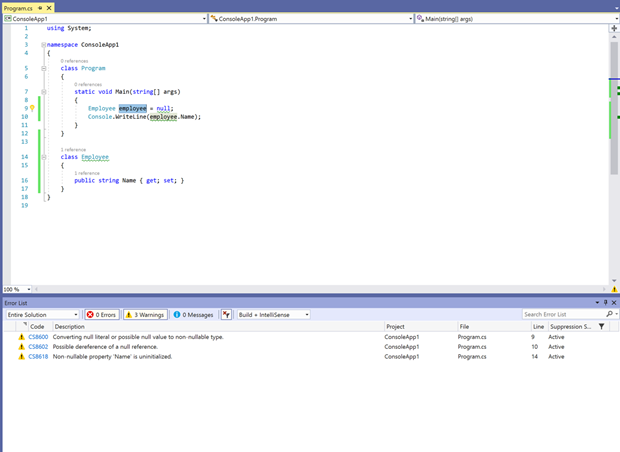 |
C# 8.0 (Visual Studio 16.0.0 Preview 1.0) |
I am sure you can spot the difference. Visual Studio is not issueing any warnings in the first picture, but in the second, Visual Studio is issuing a couple of warnings.
This is because C# 8.0 is introducing nullable reference types and non-nullable reference types. So starting with C# 8.0, all the reference type variables are not nullable and if the compiler sees a code trying to dereference a non-nullable reference type, it will issue a warning. But as of now, you are opted-out for this feature by default. You can opt-in to this feature (which is known as nullable contexts) using the following two approaches.
This is by adding the following line to the .csproj file right after the LanguageVersion.
<NullableReferenceTypes>true</NullableReferenceTypes>
- Using a newly introduced pragma, #nullable.
#nullable enable
#nullable disable
I am using project-wide setting for the post here.
Now if we have a look at the warnings Visual Studio complains about, it has three warnings.
CS8600: Converting null literal or possible null value to non-nullable type.
This is because our the variable employee of type Employee is no longer nullable. If we expect the variable to be nullable, we can change the variable initialization as follows (just as we will do for values types).
Employee? employee = null;
And prior to C# 8.0 this wasn’t possible.
CS8602: Possible dereference of a null reference.
Since our employee variable can be null, we need to update the code to add null conditional operator which got introduced with C# 6.
Console.WriteLine(employee?.Name);
CS8618: Non-nullable property 'Name' is uninitialized.
This is a nice one. Name property of Employee is a type of string and string is a reference type. Now in our sample, employee is null meaning that a value for Name is not set. To fix this we can update the code as follows.
public string? Name { get; set; }
And again this wasn’t possible prior to C# 8.0.
null-forgiving operator (!.)
This is another feature which is part of nullable reference types. Consider the below code.
class Program
{
static void Main(string[] args)
{
Employee? employee = null;
// some code
Console.WriteLine(employee.Name);
}
}
class Employee
{
public string? Name { get; set; }
}
This will again issue us “CS8602: Possible dereference of a null reference”. If we know employee is not going to be null because of some code, we can override the warning by updating the code as below.
Console.WriteLine(employee!.Name);
I am sure nullable reference types will tremendously help us in writing quality code.
Happy Coding.
Regards,
Jaliya