As most of you know, Visual Studio Ultimate 2015 Preview, the preview version of Microsoft’s next major release of Visual Studio is out. C# 6 is the version of C# which gets shipped with Microsoft Visual Studio 2015 Preview.
If you have installed Visual Studio 2015 Preview and if you take a look at the About, you will see that Microsoft .NET Framework version as 4.5.5.
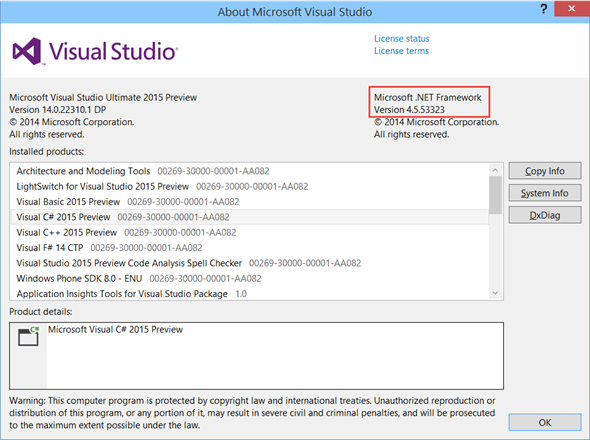 |
Visual Studio Version |
Leading up to the Preview release, it will be .NET Framework 4.5.5. Since this release includes significant features and improvements, there will be a major version increase to clearly communicate the magnitude of changes. So the next version of .NET Framework will be .NET Framework 4.6. Microsoft will be updating these in the future releases.
So let’s start getting to know what’s new in C# 6.
In brief, following are the list of new changes.
- Using static classes
- Auto property enhancements
- Initializers for auto properties
- Getter only auto properties
- Expression-bodied methods
- String Interpolation
- Index initializers
- Exception filters
- nameof expressions
- Null conditional operators
- Await in catch and finally
- Parameterless constructors in structs
Now let’s explore each of these in detail.
Using static classes
This feature allows specifying a type in a using clause, making all the accessible static members of the type available without qualification in subsequent code:
using System.Console;
class Program
{
static void Main(string[] args)
{
WriteLine("Hello C# 6.0");
}
}
Auto property enhancements
Initializers for auto properties
Now you can initialize a auto property, the moment you declared it.
public class Employee
{
public string FirstName { get; set; } = "Jaliya";
public string LastName { get; set; } = "Udagedara";
}
Getter only auto properties
In the above example, when the auto property gets initialized, it doesn’t invoke the set.
So the following is also possible.
public string LastName { get; } = "Udagedara";
But the getter-only property will of course be read-only. If you are not initializing a getter-only property value from the auto property initializer, the next and the last place you can do so is from the types constructor as follows.
public class Employee
{
public string FirstName { get; set; } = "Jaliya";
public string LastName { get; }
public Employee(string lastName)
{
this.LastName = lastName;
}
}
Expression bodied methods
Now you can use the lambda operator to modify the property in the following way.
public class Employee
{
public string FirstName { get; set; } = "Jaliya";
public string LastName { get; }
public DateTime DateOfBirth { get; set; }
public int Age => DateTime.Now.Year - DateOfBirth.Year;
public Employee(string lastName)
{
LastName = lastName;
}
}
In the previous versions of C#, to set up the value for the Age, you will have to write a getter-only property and inside the get, you need to write the logic. But now you can do it inline.
You also can use the lambda operator to define a method as follows.
public override string ToString() => string.Format("{0} {1}", FirstName, LastName);
String interpolation
With the introduction of string interpolation, you don’t have to write string.Format. You can pass your variables directly as follows and behind the scene, compiler will call the string.Format for you.
public override string ToString() => "\{FirstName} \{LastName}";
Index Initializers
Previous versions of C# has collection initializers as well as object initializers. Now you have index initializers as well. Please take a look at the following static helper method which returns a Dictionary<int,Employee>.
public static Dictionary<int, Employee> GetEmployees()
{
return new Dictionary<int, Employee>()
{
[0] = new Employee() { FirstName = "Jaliya" },
[1] = new Employee() { FirstName = "John" },
[2] = new Employee() { FirstName = "Jane" }
};
}
Exceptional filters
I have the following Main method. There I have the List of Employees which I have initialized to null;
static void Main(string[] args)
{
List<Employee> employees = null;
}
Now let’s say, mistakenly I am trying to display the count of the employees list. I should be getting an NullReferenceException. So now starting with the C# 6, you can add a exception filter as follows.
static void Main(string[] args)
{
List<Employee> employees = null;
try
{
WriteLine(employees.Count);
}
catch (Exception ex) if (ex is NullReferenceException)
{
throw;
}
catch (Exception ex)
{
}
}
If the exception is a NullReferenceException, first catch block will run. Otherwise, it will flow to the super type catch exception.
nameof
In the above piece of code, rather than throwing the exception, you will want to throw an NullReferenceException with the name of the the null argument.
static void Main(string[] args)
{
List<Employee> employees = null;
try
{
WriteLine(employees.Count);
}
catch (Exception ex) if (ex is NullReferenceException)
{
throw new NullReferenceException(nameof(employees));
}
catch (Exception ex)
{
}
}
So rather than typing a parameter name as a string (which is error prone), you can use the nameof expression.
Null conditional operator
With this handy new null conditional operator, you can fix the above code without throwing an error as follows.
WriteLine(employees?.Count);
If the employees is not null, then only the Count operation will be executed. You can use the null coalescing operator (introduced with C# 2.0) along with it to make it look nicer, specifying the default value if employees is null as well.
WriteLine(employees?.Count ?? 0);
And when the employees is null, the output will be 0.
Await in catch and finally blocks
The versions prior than C# 6, does not allow you to use await in catch and finally blocks. From C# 6, following is possible.
public async Task ThrowMeAnError()
{
try
{
int i = 0;
Console.WriteLine(10 / i);
}
catch (Exception)
{
await LogAsync();
}
finally
{
await LogAsync();
}
}
async static Task LogAsync()
{
await Task.Delay(1000);
}
Parameterless constructors in structs
From C# 6, you can now have parameterless constructors in structs.
struct Employee
{
public string FirstName { get; set; }
public string LastName { get; set; }
public Employee() : this("Jaliya", "Udagedara")
{
}
public Employee(string firstName, string lastName)
{
this.FirstName = firstName;
this.LastName = lastName;
}
}
So that’s it. You can find the status of these features from the following link.
Languages features in C# 6 and VB 14
I have uploaded the sample code to my OneDrive as well.
Happy Coding.
Regards,
Jaliya