Imagine your web application is having some issues and you have configured the application to show custom error messages instead of real exceptions. So one way of knowing what’s happening is, to change the web.config to throw real exceptions instead of custom error messages. And I am sure, your site viewers definitely not going to like it. So is there any other way, that we can use to see what has happened apart from using some logging? There is.
IntelliTrace can be quite useful in such cases and in this post let’s see how we can use IntelliTrace to collect information of an ASP.NET application running on IIS. So without much information let’s dive in to see this in action.
I have created a MVC application and I am having that application running on my local IIS. Currently it is working perfectly well. I can login and navigate around.
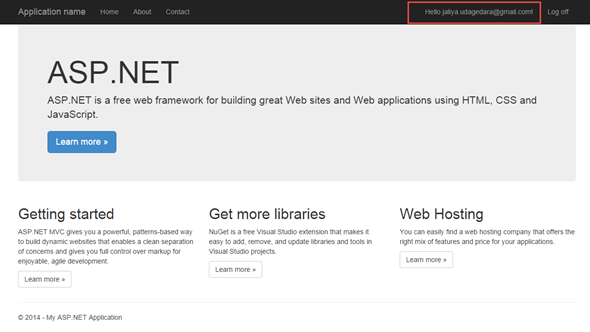 |
ASP.NET Application Running on IIS |
Now to introduce a bug, I am going to change one of the columns which is related to login functionality. So in the applications’ database, I am going to change the column “UserName” to “User_Name” in the AspNetUsers table.
 |
Changing Table Columns to Produce a Bug |
Now I am trying to login to the site back.
 |
Error |
As expected, I am getting an error. The error message is not so informative and If I don’t know a column in the database is changed, I definitely am in trouble, figuring what went wrong here. Now let’s see how I can use IntelliTrace to find out what has happened.
First you need to download and extract IntelliTrace Collector which you can download from
here. This exe is being updated every 3 months by Microsoft and it is recommended to use the latest every time. I am creating a folder named “ITraceCollector” in my C: drive and I am placing the downloaded executable there. Next what I need to do is run the executable.
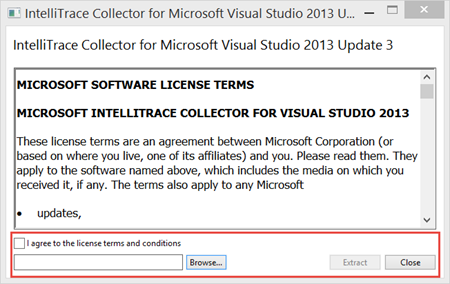 |
IntelliTraceCollector |
You need to agree on the license terms and conditions and browse for an folder to extract the content (here, after the extraction the only file I will be getting is IntelliTraceCollection.cab file). Here I am giving my extraction destination as,
C:\Program Files (x86)\Microsoft Visual Studio 12.0\Common7\IDE\CommonExtensions\Microsoft\IntelliTrace\12.0.0
One thing to note is, if you have Visual Studio installed, based on your version of Visual Studio you can find the already extracted content in above mentioned folder (if you are using Visual Studio 2012, the path would be “C:\Program Files (x86)\Microsoft Visual Studio 11.0\Common7\IDE\CommonExtensions\Microsoft\IntelliTrace\11.0.0”). When the Visual Studio is installing, it will place a IntelliTraceCollection.cab file there, but it is not the latest. So again the recommendation is to use the latest. Since I don’t want the latest IntelliTraceCollection.cab to be in a separate folder, I am just giving the original path as my extraction path.
Once the extraction is done, copy and paste the new IntelliTraceCollection.cab file to “ITraceCollector” folder which I have created before. Next I need to open up the command prompt as Administrator and run the following command to extract the content of IntelliTraceCollection.cab file.
 |
Expand IntelliTraceCollection.cab File |
cd C:\ITraceCollector
expand IntelliTraceCollection.cab F:* c:\ITraceCollector
Above what I have done is, I am changing the current directory to “c:\ITraceCollector” and I am issuing a expand command. First parameter (IntelliTraceCollection.cab) is the cab file to be expanded, second parameter (F:*) specifies all files in the cab file should be expanded. Third and the final parameter (c:\ITraceCollector) is the destination.
Once the command got completed, I can see a whole bunch of folders and files created in “C:\ITraceCollector” folder. The next thing I am interested is the default collection plan for ASP.NET applications which can be found in the “c:\ITraceCollector” folder. I need to create full collection plan and I am going modify the existing default collection plan and save as a new plan. For that download and install the “
IntelliTrace Collection Plan Configurator v1” from
here. Once installed, open up the “
IntelliTrace Collection Plan Configurator v1”.
Go to File –> Open.
 |
IntelliTrace Collection Plan Configurator |
Select the “collection_plan.ASP.NET.default.xml”
 |
Opening Default ASP.NET Collection Plan |
Once the selected collection plan is opened, go to “IntelliTrace Events” tab and select all. This will cause the collection process we are planning to run to collect all the information of all the IntelliTrace events.
 |
Selecting All IntelliTrace Events |
Once that is done, let’s go ahead with saving the file and I am giving a new name as “collection_plan_full.xml”.
 |
collection_plan_full.xml |
Now the next thing be done is initiate the IntelliTrace collection process. For that let’s open up the PowerShell as Administrator.
First I am importing the PowerShell module for IntelliTrace. You can find it “C:\ITraceCollector” folder.
 |
Importing IntelliTrace Module |
Import-Module c:\ITraceCollector\Microsoft.VisualStudio.IntelliTrace.PowerShell.dll
Now let’s check what are the available IntelliTrace commands by running Get-Help command.
 |
Get IntelliTrace Help |
There I have a command named “
Start-IntelliTraceCollection”. I am issuing that command.
 |
Starting IntelliTrace Collection |
It is asking for name of Application Pool. I am getting the Application Pool by going to IIS, right clicking on my application and Manage Application –> Advanced Settings.
 |
Application Properties |
 |
Getting Application Pool Name |
I am copying the Application Pool name which is “DefaultAppPool” and pasting it in the PowerShell.
 |
Application PoolName |
Then it is asking me for the collection plan. I have created a full collection plan before and I am giving the path of that particular xml file which is “C:\ITraceCollector\collection_plan_full.xml”.
 |
Collection Plan Name |
Now it’s asking for the Output path where trace files should be created. For that I am creating a folder named “ITraceLogFiles” in my C: drive and I am giving that path and hitting on enter.
 |
Output Path |
I am thrown with an error “The following application pool requires write permissions to the output directory: "NTAUTHORITY\NETWORK SERVICE", "C:\ITraceLogFiles".”. Now let’s give full control to the folder “C:\ITraceLogFiles” for the user “NTAUTHORITY\NETWORK SERVICE”.
 |
Setting Permission to ITraceLogFiles Folder |
After doing that, let’s run Start-IntelliTraceCollection command back again and it’s time let’s make it short by issuing the command from one line.
Start-IntelliTraceCollection "DefaultAppPool" c:\ITraceCollector\collection_plan_full.xml c:\IntelliTraceLogFiles
 |
Start-IntelliTraceCollection |
No errors this time and it’s asking me to confirm the action. I am entering “Y”.
Now, IntelliTrace collection process has been started. Now let’s try to log in to my web application back again so those events will be collected by IntelliTrace.
 |
Error |
Again, I am getting the error. That’s fine and let’s examine the “C:\ITraceLogFiles”.
 |
Created iTrace Files |
I can see a iTrace file created there. Since now I know my actions on the web application has been traced by IntelliTrace, let’s stop the IntelliTrace collection by issuing Stop-IntelliTraceCollection command. Now it is asking for the Application pool and I am giving it as “DefaultAppPool” and like previous, I am confirming the action.
 |
Stop-IntelliTraceCollection |
Again you can do this by issuing following command in one line.
Stop-IntelliTraceCollection DefaultAppPool
Since IntelliTrace collection is now stopped, let’s open the iTrace file using Visual Studio. You just have to double click on that file, it will be opened using Visual Studio.
 |
IntelliTrace Events |
After scrolling a bit I can see several events logged saying “Invalid column name ‘UserName’”. And when I double clicked on an event, it starts to debug with the IntelliTrace window.
 |
Debug Mode |
Since the error is thrown from ASP.NET Identity, I cannot debug the code here. If an error is thrown in code written by me, I should be able to directly navigate to the code.
So that’s all and hope you would found this interesting.
Happy Coding.
Regards,
Jaliya