From Azure Functions, we can map data to Output bindings using following three options.
Using function return value
Using out parameter
Using ICollector or IAsyncCollector
In this post let’s have a look at them in detail. I have created a Azure Function App using the Azure Portal and I will be using portals’ editor in this post.
The scenario I am going use is, I will have a Azure Function which can be triggered manually. From there I will be outputting messages to a Azure Storage Queue.
So first let’s create a Queue trigger.
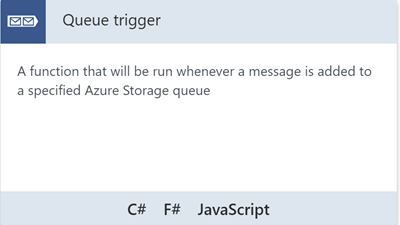 |
Queue trigger |
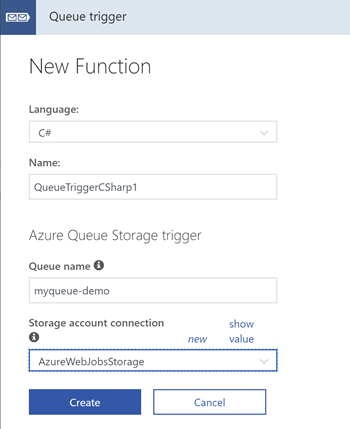 |
Queue trigger Properties |
using System;
public static void Run(string myQueueItem, TraceWriter log)
{
log.Info($"Queue trigger function processed: {myQueueItem}");
}
So every time a message get queued to myqueue-demo, QueueTriggerCSharp1 function will get triggered. It will just log the message, so we can see whether the data is getting correctly mapped when we are using Output bindings in our Manual trigger.
Now let’s create the Manual trigger.
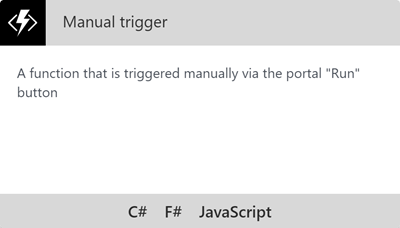 |
Manual trigger |
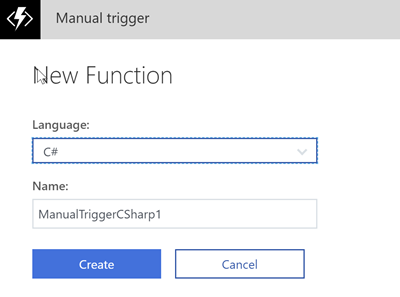 |
Manual trigger Properties |
using System;
public static void Run(string input, TraceWriter log)
{
log.Info($"Manually triggered function called with input: {input}");
}
1. Using function return value
The most easiest way to map data to Output binding is using the functions return value.
I am changing our ManualTriggerCSharp1 as follows.
using System;
public static string Run(string input, TraceWriter log)
{
log.Info($"Manually triggered function with input: {input}");
return $"Using Return: {input}";
}
Now we have a function which returns a string. Our requirement is map this return value to a Output binding.
For that, let’s go to Integrate menu under our ManualTriggerCSharp1 function.
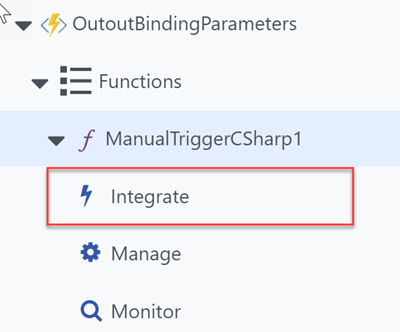 |
Integrate |
And you will see something like below.
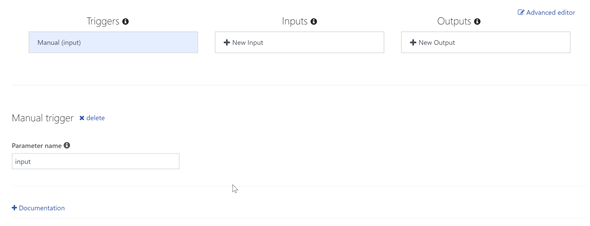 |
Integrate |
Click on New Output under Outputs. Select Azure Queue Storage.
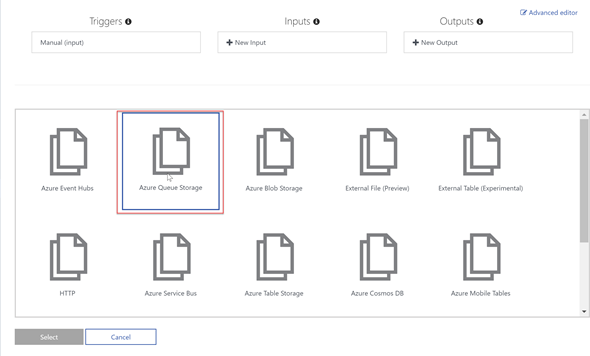 |
Select Output Type |
From next wizard page, you can simply select the “Use function return value”. Then you will need to select the Queue name and it’s Storage account connection which you want to write the output into.
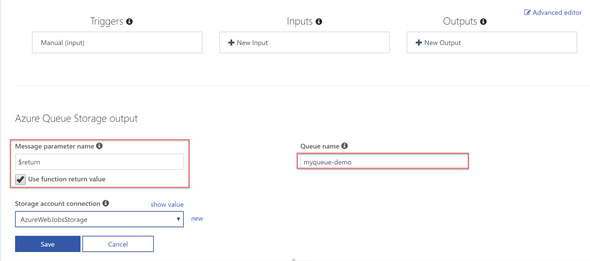 |
New Output Properties |
Click on Save, and run our Manual trigger. After running it, if you go to Monitor menu under QueueTriggerCSharp1, you can see the return value has been read.
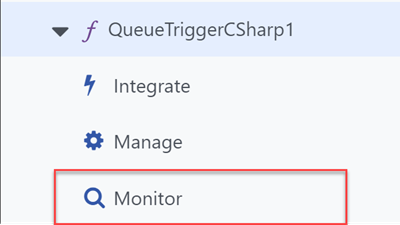 |
Monitor |
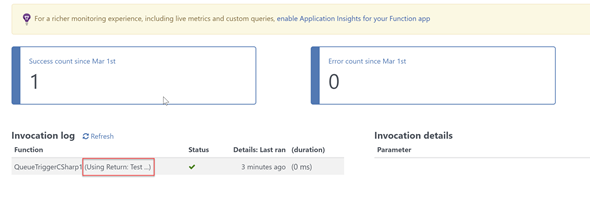 |
Monitor |
2. Using out parameter
Now let’s modify our ManualTriggerCSharp1 function to have out parameter and set it’s value in the function body.
using System;
public static string Run(string input, TraceWriter log, out string outParameter)
{
log.Info($"Manually triggered function with input: {input}");
outParameter = $"Using Out: {input}";
return $"Using Return: {input}";
}
Now let’s add this Output binding.
Again add a New Output and select Azure Queue Storage and then do as following (remember to specify the correct Queue name which our QueueTriggerCSharp1 is watching).
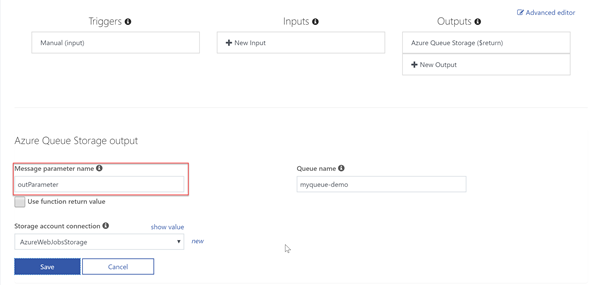 |
New Output Properties |
Important thing to keep in mind is Message parameter name needs to be same as your out parameter name.
Save and run the manual trigger and examine the Monitor menu under QueueTriggerCSharp1.
Now you should be seeing the value of out parameter has been added to queue and is read.
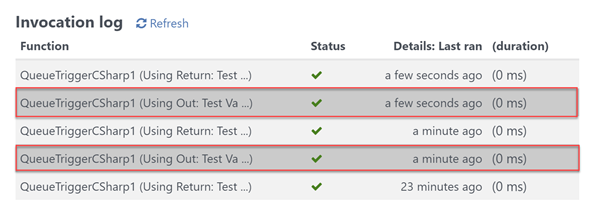 |
Monitor |
Now what if the function is an async one. Then we can’t use out parameters.
3. Using ICollector or IAsyncCollector
This is my preferred way of mapping data to Output bindings. One reason of course is most of the time, functions are async. And the other reason is, using Collectors we can pass multiple data. Let’s modify the ManualTriggerCSharp1 as follows.
using System;
public static async Task<string> Run(string input, TraceWriter log, IAsyncCollector<string> queueCollector)
{
log.Info($"Manually triggered function with input: {input}");
await queueCollector.AddAsync($"Using Collector: {input} 1");
await queueCollector.AddAsync($"Using Collector: {input} 2");
return $"Using Return: {input}";
}
Right now since we don’t have the existing outParameter and it’s binding, let’s change the previous Output binding.
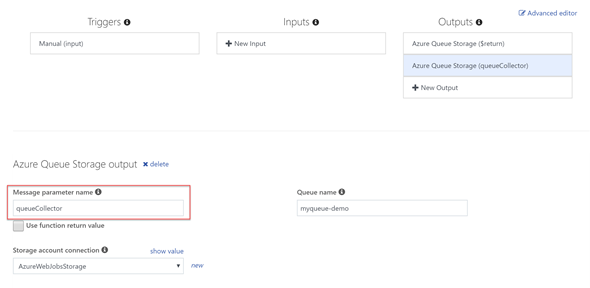 |
Output Properties |
Again, we need to make sure Message parameter name is same as the function parameter name.
And if we save and run, we should be seeing the message is queued and read by QueueTriggerCSharp1.
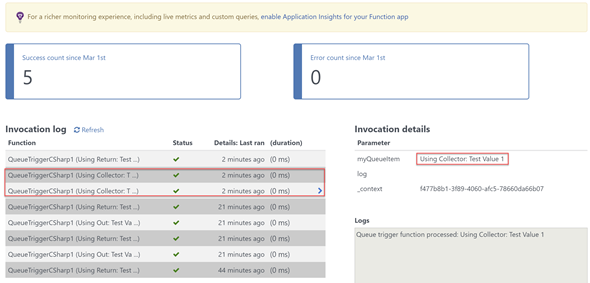 |
Monitor |
And note: ICollector is the synchronous counterpart of IAsyncCollector and I don’t think it deserves a demonstration of it’s use.
Hope this helps.
Happy Coding.
Regards,
Jaliya